A taste of Google App Script (2 of 2)
2020-08-12In previous article (A taste of Google App Script (1 of 2)), I have covered some basics of Google App Script as WebApp. Here, I’ll share some problems I’ve encountered.
Log message to console
Loggin is very important for developers to trace how the program works. Depends on where the code executes, logging is slightly different.
For server code
Logger.log("debug message");
can be used in code, and location of the outputs depend on how you execute the code.
If you execute the code via the code editor, output messages can be found from editor's menu View
▶ Logs
.
If code execution is outside of the editor, e.g. called by browser via google.script.run.functionToDebug()
, output message is located in dashboard which can also be accessed via editor's menu item View
▶ Executions
.
To know more about advanced logging setup, check the Stackdriver section from Google App Script documentation.
For browser code
Similar to other Web App development, console.log("debug message");
can be used and observe the message in developer console, e.g. F12
of Google Chrome.
Modify URL of deployed WebApp
Can I have an nice URL?
Do I have control over the URL?
etc.
The short answer is no.
Alternatively, other web hosting services like Google Sites, Netlify or Github Pages can be used to serve the web pages that references to the contents of the the WebApp.
Serve multiple HTML pages
Since there is only one entry for get request of generated URL, one can use URL parameter to decide return different pages.
Using below server code, the url <webapp url>?page=1
in browser will get the content of page1.html
.
function doGet(e) {
switch (e.parameter.page) {
case "1":
return HtmlService.createTemplateFromFile('page1').evaluate();
break;
case "2":
return HtmlService.createTemplateFromFile('page2').evaluate();
break;
default:
// When no specific page requested, return "home page"
return HtmlService.createTemplateFromFile('index').evaluate();
break;
}
}
Pass value from server side code to client side (e.g. browser) on demand
From browser, google.script.run
can be used to execute code in server side and obtain result.
Server side code (Code.gs
)
function getMagicNumber(){
return 100;
}
Client side code (browser javascript)
function getMagicNumberFromRemote(){
function handleSuccess(data) {
console.log(data);
}
function handleFailure(e) {
console.log("ERROR");
console.log(e.message);
}
google.script.run.
withSuccessHandler(handleSuccess).
withFailureHandler(handleFailure).
getMagicNumber();
}
Pass BLOB from server side
As of the time of writing (and since 2016 as on Stackoverflow), passing BLOB directly is not working and resulting an error.
The workaround is to encode the BLOB with base 64 encoding and send as string as in this Stackoverflow.com answer.
Server side code (Code.gs
)
function getBlobInBase64(fileId){
// omit authorization code if any
var file = DriveApp.getFileById(fileId);
var blob = file .getBlob();
return {
file_name: file.getName(),
mime: file.getMimeType(),
b64: Utilities.base64Encode(blob.getBytes())
};
}
Client side code (browser javascript)
function getFile(fileId){
google.script.run.withSuccessHandler((data) => {
var uri = 'data:' + data.mime + ';charset=ISO-8859-1;base64,' + encodeURIComponent(data.b64);
downloadURI(uri, data.file_name);
}).withFailureHandler((err) => {
console.log(err);
}).getBlobInBase64();
}
However, since there is no document about this, things may changed.
Include external JS library
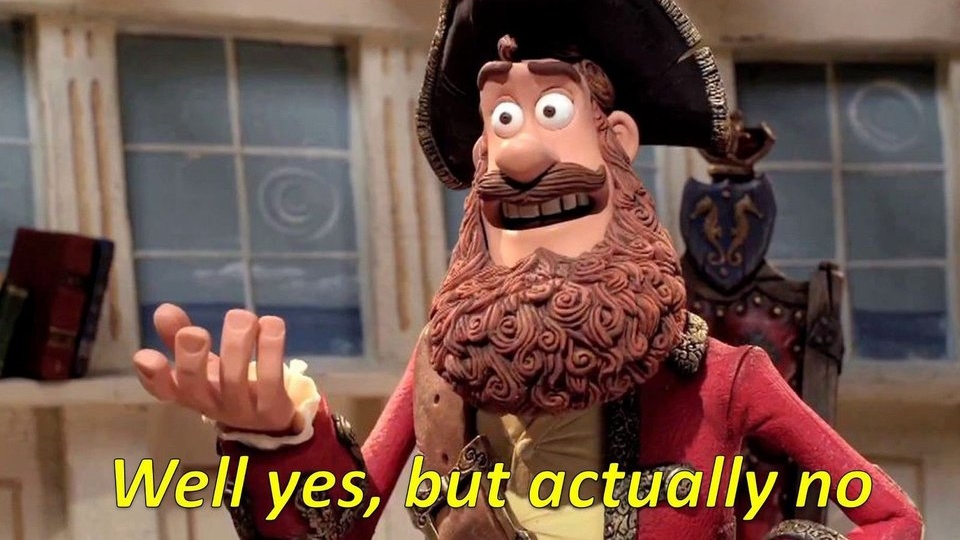
For npm packages, they cannot be used since Google App Script is not a node.js environment.
For vanilla JS libraries, theorically, eval()
, or even copy and paste the source code as a .gs
file, can be used. However, those may depends on browser functions that also not provided by Google App Script environment.
Closing
This is the end of this two part series about Google App Script. I hope this helps you understand more about Google App Script and use it happily.
If you like this article, remember to show your support by buy me a book.